Do you want to learn Python but you're overwhelmed and you don't know where to start? Learn with Python cheat sheets! They compress the most important information in an easy-to-digest 1-page format. Here's the new Python cheat sheet I just created-my goal was to make it the world's most concise Python cheat sheet! Download PDF The World’s Most Concise Python Cheat Sheet Read More ».
In general, code is written once in a few minutes (or hours) and then read and maintained for years to come. Ensuring that the code you write today is readable and makes sense to other engineers down the line is a Really Good Thing and you should always keep readability in mind as you’re writing code, even for small one-off scripts.
Python PEP8 style guide Cheat Sheet from jmds Python Cheat Sheet Python 3 is a truly versatile programming language, loved both by web developers, data scientists and software engineers. And there are several good reasons for that! Once you get a hang of it, your development speed and productivity will soar! This document gives coding conventions for the Python code comprising the standard library in the main Python distribution. Please see the companion informational PEP describing style guidelines for the C code in the C implementation of Python 1. This document and PEP 257 (Docstring Conventions) were adapted from Guido’s original Python Style Guide essay, with some additions.
This is an adaption of an article I wrote on the engineering wiki at Buffer, where I work as a growth hacker.
The Zen of Python

Run import this
from a python interpreter to see the official “zen of python”

PEP8
tl;dr: Whatever IDE or text editor you’re using probably has a plugin that will notify you when the code you’re writing doesn’t match these styles. Installing one was the best decision I ever made as a python programmer. (Besides reading “Dive into Python”)
Ideally, there should be One Correct Way of writing a given statement or expression. If everyone decides their own conventions for spacing, variable naming, etc. then code becomes harder to read and maintain. The PEP8 style guide is the most commonly used convention for addressing this problem in python. Written by Guido van Rossum – the creator of python – it recommends the following conventions:
- Use 4 spaces for indentation, not tabs
- A single space after every comma and between every operator:
- Two blank lines between classes and top-level function definitions, one blank line between class methods.
Imports should be on separate lines, and should be explicit:
Variables are where you give your code meaning and context. Variable names should err on the side of being long and explicit. It may take a few extra seconds to type, but it saves other engineers hours of trying to figure out what you meant. If your variable names are shorter than five characters, they’re probably too short.
P.S. They say one of the hardest problems in programming is naming things.
Comments can be super useful when there’s a lot of “magic” going on or if you’re worried that there’s a lot of logic that might be hard for a new reader to understand. But beware the out-of-date comment! Whenever you change code, be sure to change any nearby comments if they’re no longer true. Comments that contradict the code are worse than no comments.
Final Thought
It may seem like splitting hairs, especially if the code still functions regardless of whether it follows the style guide. But having clean, easy-to-read code is a huge investment in your code base and saves you from piling up mountains of technical debt down the line. If any engineer can open a file and quickly figure out exactly what’s happening and make edits, you become a much faster engineering team.
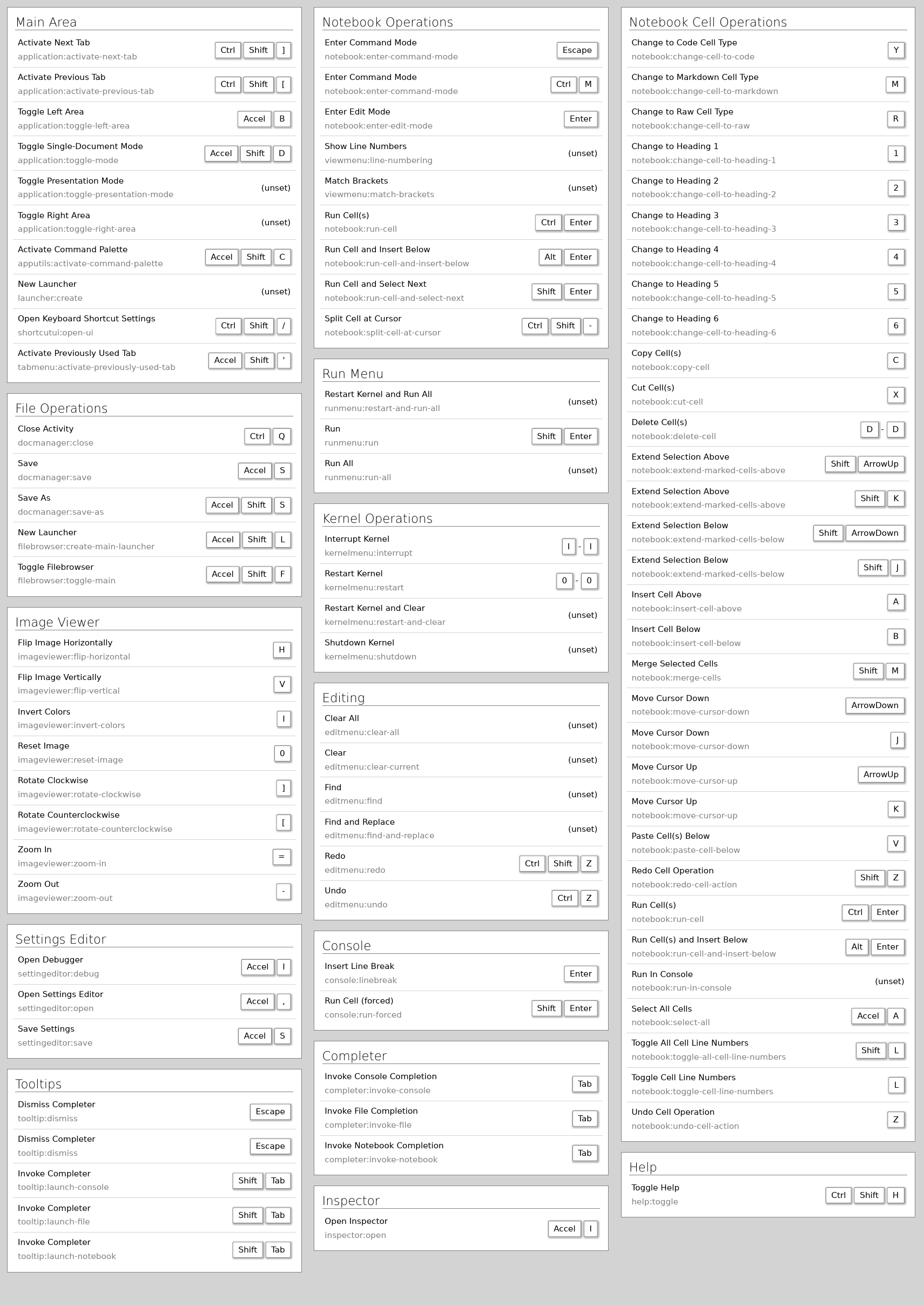
Python function arguments
Python Function Arguments, How do you pass a function as an argument in Python? Information can be passed into functions as arguments. Arguments are specified after the function name, inside the parentheses. You can add as many arguments as you want, just separate them with a comma. The following example has a function with one argument (fname).
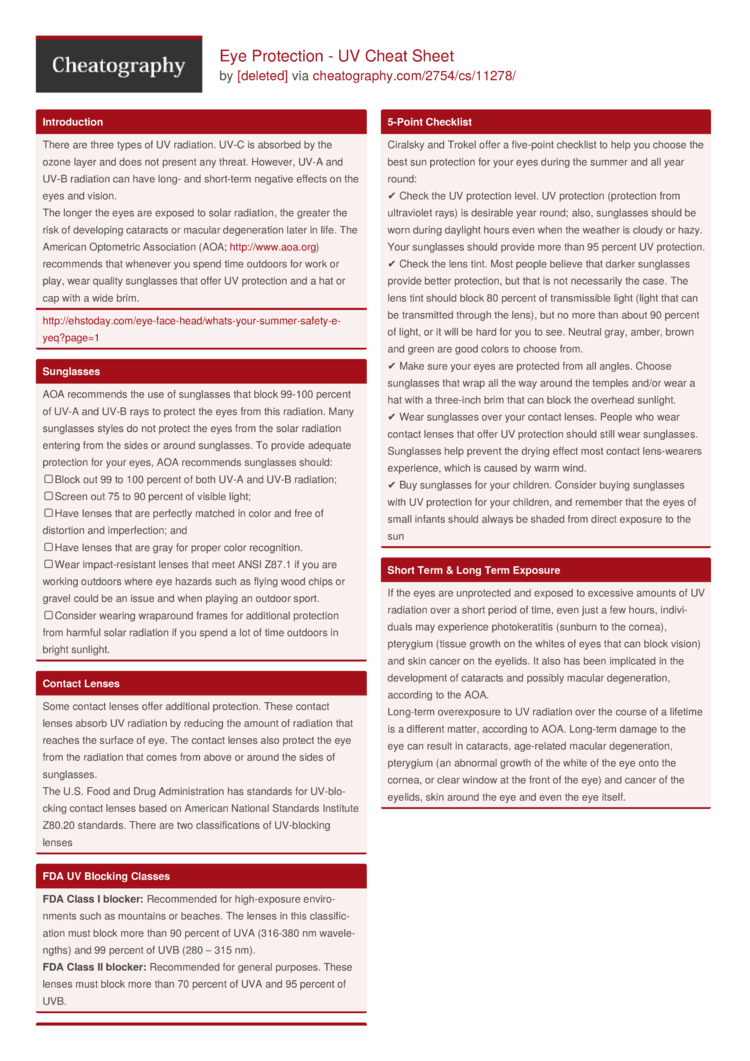
Python Function Arguments (Default, Keyword and Arbitrary), ) as a variable whose value is the actual callable code object. Python allows functions to be called using keyword arguments. When we call functions in this way, the order (position) of the arguments can be changed. Following calls to the above function are all valid and produce the same result.
How do I pass a method as a parameter in Python, refers to within a function, the change also reflects back in the calling function. Python offers a way to write any argument in any order if you name the arguments when calling the function. This allows us to have complete control and flexibility over the values sent as arguments for the corresponding parameters.
Python style guide
This document and PEP 257 (Docstring Conventions) were adapted from Guido's original Python Style Guide essay, with some additions from Barry's style guide. This style guide evolves over time as additional conventions are identified and past conventions are rendered obsolete by changes in the language itself.
The style guide originally at this URL has been turned into two PEPs (Python Enhancement Proposals): PEP 8 for the main text, and PEP 257 for docstring conventions.
PEP 8 is the de facto code style guide for Python. A high quality, easy-to-read version of PEP 8 is also available at pep8.org. This is highly recommended reading. The entire Python community does their best to adhere to the guidelines laid out within this document.
Python function arguments list
Python Passing a List as an Argument, You can send any data types of argument to a function (string, number, list, dictionary etc.), and it will be treated as the same data type inside the function. Arguments are specified after the function name, inside the parentheses. You can add as many arguments as you want, just separate them with a comma. The following example has a function with one argument (fname). When the function is called, we pass along a first name, which is used inside the function to print the full name:
Call a function with argument list in python, To expand a little on the other answers: In the line: def wrapper(func, *args):. The * next to args means 'take the rest of the parameters given Python Program arguments can have default values. We assign a default value to an argument using the assignment operator in python (=). When we call a function without a value for an argument, its default value (as mentioned) is used. >>> def greeting (name='User'): print (f'Hello, {name}') >>> greeting ('Ayushi')
Multiple Function Arguments - Learn Python, It is possible to declare functions which receive a variable number of arguments, using the following syntax: · The 'therest' variable is a list of variables, which Passing a List as an Argument. You can send any data types of argument to a function (string, number, list, dictionary etc.), and it will be treated as the same data type inside the function. E.g. if you send a List as an argument, it will still be a List when it reaches the function:
Pep8
This stylized presentation of the well-established PEP 8 was created by Kenneth Reitz (for humans). Introduction This document gives coding conventions for the Python code comprising the standard library in the main Python distribution.
A Foolish Consistency is the Hobgoblin of Little Minds. One of Guido's key insights is that code is read much more often than it is written. The guidelines provided here are intended to improve the readability of code and make it consistent across the wide spectrum of Python code.
PEP 8, sometimes spelled PEP8 or PEP-8, is a document that provides guidelines and best practices on how to write Python code. It was written in 2001 by Guido van Rossum, Barry Warsaw, and Nick Coghlan. The primary focus of PEP 8 is to improve the readability and consistency of Python code.
PEP 8 coding style constant values
PEP 8 -- Style Guide for Python Code, If you are going to treat the value as a constant in the rest of your code, by all means, use CONSTANT_CASE for those globals. It's up to you, Please see the companion informational PEP describing style guidelines for the C code in the C implementation of Python . This document and PEP 257 (Docstring Conventions) were adapted from Guido's original Python Style Guide essay, with some additions from Barry's style guide [2] .
When is a variable considered constant in terms of PEP8 naming , This tutorial outlines the key guidelines laid out in PEP 8. Other people, who may have never met you or seen your coding style before, will have to read and Constant, Use an uppercase single letter, word, or words. Note: When = is used to assign a default value to a function argument, do not surround it with spaces. Please see the companion informational PEP describing style guidelines for the C code in the C implementation of Python 1. This document and PEP 257 (Docstring Conventions) were adapted from Guido’s original Python Style Guide essay, with some additions from Barry’s style guide 2 .
How to Write Beautiful Python Code With PEP 8 – Real Python, more than one custom value accessor matches form control with unspecified name attributerails model naming conventionmanually validate classlaravel >>> A = 2 # a declared constant >>> B = 2 # another declared constant >>> TOTAL = A + B # 'caps' per PEP8 for constant naming 4 Assuming the program has terminated and TOTAL is never altered, I would consider this value a constant. Again under the presumption that any variable that is not alterable during runtime is to be considered a constant.
Python style guide Google
styleguide, Python is the main dynamic language used at Google. This style guide is a list of dos and don'ts for Python programs. To help you format code correctly, we've Python is the main dynamic language used at Google. This style guide is a list of dos and don’ts for Python programs. To help you format code correctly, we’ve created a settings file for Vim. For Emacs, the default settings should be fine.
Example Google Style Python Docstrings, coding: utf-8 -*- ''Example Google style docstrings. _Google Python Style Guide: http://google.github.io/styleguide/pyguide.html '' module_level_variable1 To enable screen reader support, press Ctrl+Alt+Z To learn about keyboard shortcuts, press Ctrl+slash Whenever you write Python code for this class, follow the guidelines below, adapted from the
Google Code Archive, soc - PythonStyleGuide.wiki. Melange follows the Google Python Style Guide; this document describes only those respects in which Melange's style differs from Google Python Style Guide. 1 Background. Python is the main dynamic language used at Google. This style guide is a list of dos and don'ts for Python programs. To help you format code correctly, we've created a settings file for Vim. For Emacs, the default settings should be fine. Many teams use the yapf auto-formatter to avoid arguing over
Python function name convention
Python Naming Convention. The style guide for Python is based on Guido’s naming convention recommendations. Functions: lower_with_under() _lower_with_under()
Python naming conventions for variable names are same as function names. There are some rules we need to follow while giving a name for a Python variable . Rule-1 : You should start variable name with an alphabet or underscore(_) character.
Function names should be lowercase, with words separated by underscores as necessary to improve readability. Variable names follow the same convention as function names. mixedCase is allowed only in contexts where that's already the prevailing style (e.g. threading.py), to retain backwards compatibility.
Python optional arguments
Python Optional Arguments: A How-To Guide, when you call a function. This is because the default value will be used if one is not specified. A Python optional argument is an argument with a default value. You can specify a default value for an argument using the assignment operator. There is no need to specify a value for an optional argument when you call a function. This is because the default value will be used if one is not specified.
How do I create a Python function with optional arguments?, Try calling it like: obj.some_function( '1', 2, '3', g='foo', h='bar' ) . After the required positional arguments, you can specify specific optional The arguments d through h are strings which each have different meanings. It is important that I can choose which optional parameters to pass in any combination. For example, (a, b, C, d, e), or (a, b, C, g, h), or (a, b, C, d, e, f, or all of them (these are my choices).
Python Function Arguments (Default, Keyword and Arbitrary), How to create functions with optional arguments in Python. Office mac 2019 torrent. Use default values. Use the *args parameter. Functions can have required and optional arguments. You can make any number of arguments or parameters optional in python. To make an argument optional, you have to assign some default value to that argument. Here, to make the age argument optional, you can add a default value to the argument age in the function definition to make it optional.
Argument Python
Python Function Arguments, Python provides a getopt module that helps you parse command-line options and arguments. $ python test.py arg1 arg2 arg3. The Python sys module provides Information can be passed into functions as arguments. Arguments are specified after the function name, inside the parentheses. You can add as many arguments as you want, just separate them with a comma. The following example has a function with one argument (fname).
Python - Command Line Arguments, The ArgumentParser object will hold all the information necessary to parse the command line into Python data types. Adding arguments¶. Filling an In Python, there are other ways to define a function that can take variable number of arguments. Three different forms of this type are described below. Python Default Arguments. Function arguments can have default values in Python. We can provide a default value to an argument by using the assignment operator (=). Here is an example.
argparse, Arguments. The arguments are also called operands or parameters in the POSIX standards. The arguments represent the source or the The Python sys module provides access to any command-line arguments via the sys.argv. This serves two purposes − sys.argv is the list of command-line arguments. len (sys.argv) is the number of command-line arguments.
Error processing SSI filePython class
9. Classes, Python classes provide all the standard features of Object Oriented Programming: the class inheritance mechanism allows multiple base classes, a derived class can override any methods of its base class or classes, and a method can call the method of a base class with the same name. Next Dates & Prices. Live Instructors Teaching. Python Class. Attend From: Home/Work, Computer Lab or Onsite.
Python Classes, A class is a user-defined blueprint or prototype from which objects are created. Classes provide a means of bundling data and functionality Enhance Your Python Skills With Expert-Led Online Video Tutorials - Start Now!
Python Classes and Objects [With Examples], Objects get their variables and functions from classes. Classes are essentially a template to create your objects. A very basic class would look something like this Learn Python Like a Pro. From The Basics All The Way to Creating your own Apps and Games! Join Over 30 Million Students Already Learning Online With Udemy
Python Pep8 Cheat Sheet
Error processing SSI filePython variable naming conventions
Naming Styles, Use a lowercase single letter, word, or words. Separate words with underscores to improve readability. Python does not support privacy directly. This naming convention is used as a weak internal use indicator. Should follow the above naming conventions Should use a leading underscore (_) to distinguish between 'public' and 'private' variables
Pep8 Cheat Sheet Pdf
PEP 8 -- Style Guide for Python Code, Start each word with a capital letter. Do not separate words with underscores. This style is called camel case. Python naming conventions for variable names are same as function names. There are some rules we need to follow while giving a name for a Python variable . Rule-1 : You should start variable name with an alphabet or underscore(_) character.
What is the naming convention in Python for variable and function , How to Write Beautiful Python Code With PEP 8 – Real Python Python Naming Convention. The style guide for Python is based on Guido’s naming convention recommendations. List of covered sections: Class Naming; Constant Naming; Method Naming; Module Naming; Variable Naming; Package Naming; Exception Naming; Underscore; TL;DR
Error processing SSI filePython naming conventions cheat sheet
How to Write Beautiful Python Code With PEP 8 – Real Python, Naming Conventions. “Explicit is better than implicit.” — The Zen of Python. When you write Python code, you have to name a Python Naming Convention. The style guide for Python is based on Guido’s naming convention recommendations. List of covered sections:
Python PEP8 style guide Cheat Sheet by jmds, Notice that this in not PEP8-cheatsheet.py See http://www.python.org/dev/peps/pep-0008/ for more PEP-8 details. '' Common naming convention names:. instead of accessor functions to avoid the extra cost of function calls in Python. When more functionality is added you can use property to keep the syntax consistent. Naming. module_name, package_name, ClassName, method_name, ExceptionName, function_name, GLOBAL_CONSTANT_NAME, global_var_name, instance_var_name, function_parameter_name, local
PEP 8: The Style Guide for Python Code, packages should also have short, all-lowercase names, although the use of underscores is discouraged. The naming conventions of Python's library are a bit of a mess, so we'll never get this completely consistent -- nevertheless, here are the currently recommended naming standards. New modules and packages (including third party frameworks) should be written to these standards, but where an existing library has a different style, internal
Error processing SSI filePep8 Standards Cheat Sheet
Python Pep8 Cheat Sheet Pdf
More Articles
